I've worked out how to do this in an AwareIM Query "Initialization script". The example below is for a Query that has 3 columns of phone numbers that are stored as strings with no spaces.
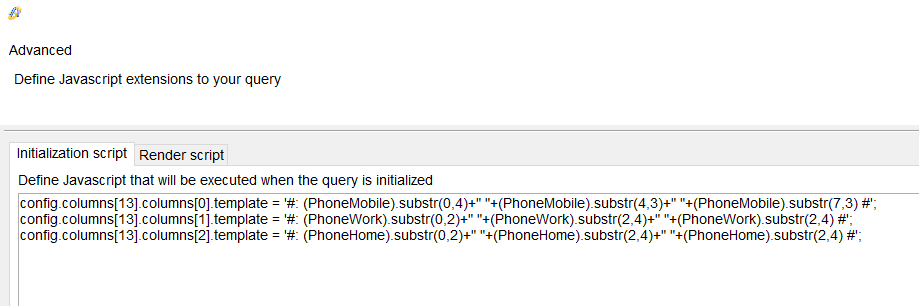
This is the code if you want to copy it.
config.columns[13].columns[0].template = '#: (PhoneMobile).substr(0,4)+" "+(PhoneMobile).substr(4,3)+" "+(PhoneMobile).substr(7,3) #';
config.columns[13].columns[1].template = '#: (PhoneWork).substr(0,2)+" "+(PhoneWork).substr(2,4)+" "+(PhoneWork).substr(2,4) #';
config.columns[13].columns[2].template = '#: (PhoneHome).substr(0,2)+" "+(PhoneHome).substr(2,4)+" "+(PhoneHome).substr(2,4) #';
Note that there are 2 references to "columns" because this Query has grouped columns like below :
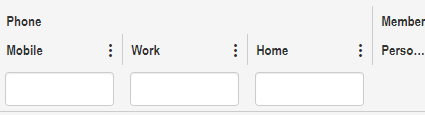
If this Query did not have grouped columns the code would be
config.columns[10].template = '#: (PhoneMobile).substr(0,4)+" "+(PhoneMobile).substr(4,3)+" "+(PhoneMobile).substr(7,3) #';
config.columns[11].template = '#: (PhoneWork).substr(0,2)+" "+(PhoneWork).substr(2,4)+" "+(PhoneWork).substr(2,4) #';
config.columns[12].template = '#: (PhoneHome).substr(0,2)+" "+(PhoneHome).substr(2,4)+" "+(PhoneHome).substr(2,4) #';
When you use this you will need to change the number of the column
[10]
to match your column sequence and the name of the AwareIM attribute
PhoneMobile
to match your own AwareIM attribute name. Note you can use this to put anything into any column including HTML tags to get different effects. The Query filters use the original data so searching when a phone number is stored as 1212341234 and renders as 12 1234 1234 you need to search WITHOUT spaces.
Note also you can call javascript methods (like substr() in the first example above) OR you own javascript and kendo functions. I tried to call kendo functions to use a mask but failed so I have ended up making this javascript function.
function usKendoMask(value,mask) {
// Applies and returns a Kendo "mask"ed "value"
// Example results
// value 0212341234 mask 99 0000 0000 result 02 1234 1234
// value 12341234 mask 99 0000 0000 result 1234 1234
// value 0212341234 mask 99-0000-0000 result 02-12341234
var result='';
if ((typeof(value)!='undefined') && value!='') {
var pnt=value.length - 1;
for (var i = mask.length - 1; i >= 0; i--){
if (mask[i]=='0') {
result=value[pnt]+result;
pnt-=1;
} else if (mask[i]=='9' && pnt>=0) {
result=value[pnt]+result;
pnt-=1;
} else {
result=mask[i]+result;
}
}
}
return result;
}
}
So now I 'm using the following code in the "Initialization script".
config.columns[13].columns[0].template = '#: usKendoMask(PhoneMobile,"0000 000 000") #';
config.columns[13].columns[1].template = '#: usKendoMask(PhoneWork,"99 0000 0000") #';
config.columns[13].columns[2].template = '#: usKendoMask(PhoneHome,"99 0000 0000") #';
You can read more about the kendo templates at https://docs.telerik.com/kendo-ui/framework/templates/overview