Asked GPT:
Q:
How to add multiple action lists in kendo ui grid: one list to be as drop-down and one list to have seperate action buttons on same row?
R:
To add multiple action lists in a Kendo UI Grid, where:
- One list is a dropdown (DropDownList)
- Another list has separate action buttons in the same row>
`
$(document).ready(function() {
$("#grid").kendoGrid({
dataSource: {
data: [
{ id: 1, name: "Item 1", action: "", status: "Pending" },
{ id: 2, name: "Item 2", action: "", status: "Completed" }
],
schema: {
model: {
fields: {
id: { type: "number" },
name: { type: "string" },
action: { type: "string" },
status: { type: "string" }
}
}
}
},
columns: [
{ field: "id", title: "ID", width: "50px" },
{ field: "name", title: "Name", width: "150px" }
// 🔽 DropDown Action List Column
{
title: "Actions",
template: function(dataItem) {
return `<input class="action-dropdown" data-id="${dataItem.id}" />`;
},
width: "150px"
},
// 🔘 Separate Action Buttons Column
{
title: "Options",
template: function(dataItem) {
return `
<button class="btn-edit" data-id="${dataItem.id}">Edit</button>
<button class="btn-delete" data-id="${dataItem.id}">Delete</button>
`;
},
width: "200px"
}
],
dataBound: function() {
// Initialize DropDownList for each row
$(".action-dropdown").each(function() {
var id = $(this).data("id");
$(this).kendoDropDownList({
dataSource: ["View", "Approve", "Reject"],
optionLabel: "Select Action",
change: function(e) {
var selectedAction = this.value();
alert("Selected Action: " + selectedAction + " for ID: " + id);
}
});
});
// Handle button click events
$(".btn-edit").on("click", function() {
var id = $(this).data("id");
alert("Edit clicked for ID: " + id);
});
$(".btn-delete").on("click", function() {
var id = $(this).data("id");
alert("Delete clicked for ID: " + id);
});
}
});
});
`
You can achieve this using a combination of template columns and event handling. Below is a complete solution:
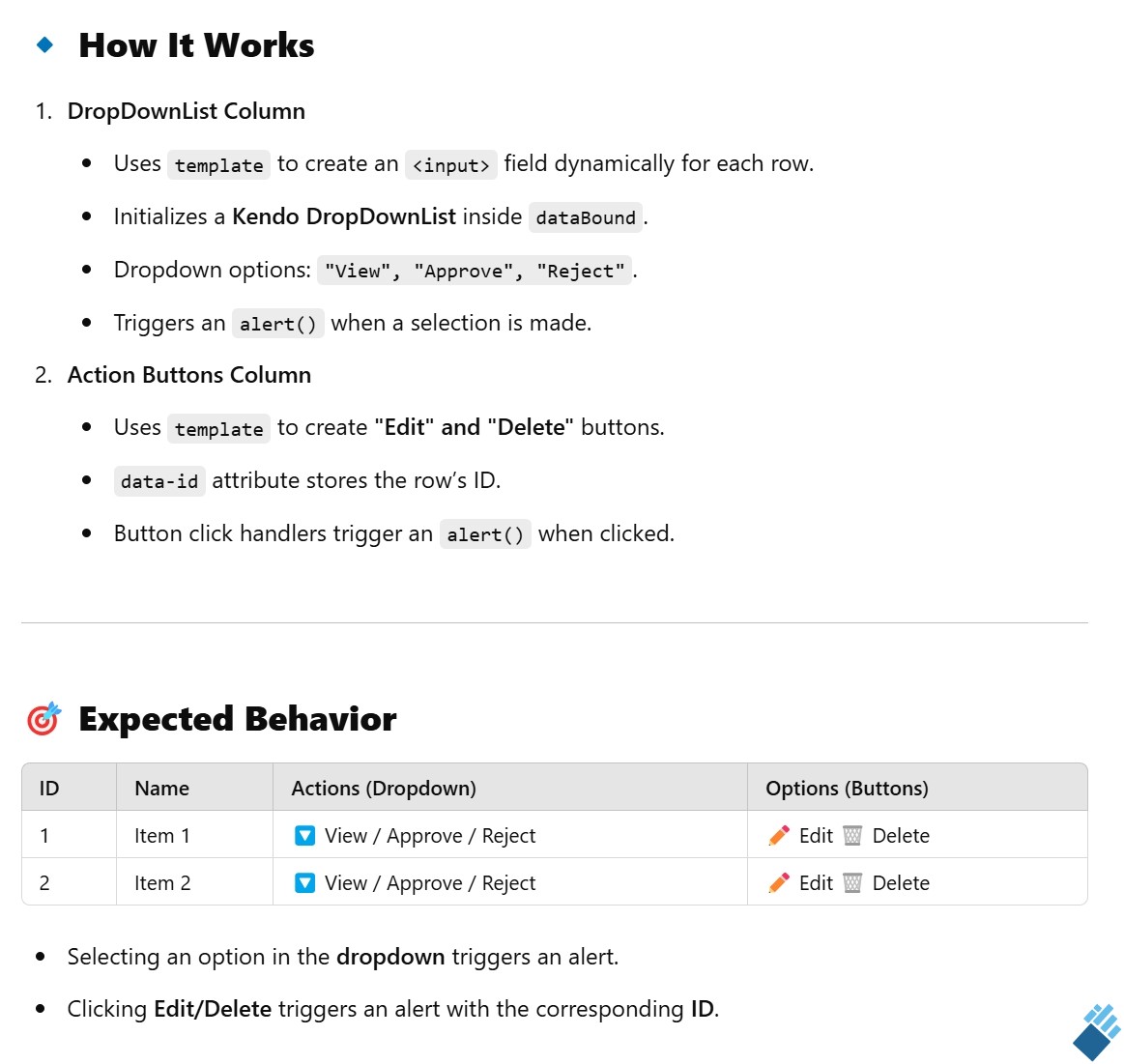